REST API
REST API allows you to access RESTful web services, allowing you to read, create, update and delete data, as supported by the service.
Authentication
Before accessing a REST API service, the user must be authenticated. OmniFi has native support for Basic and OAuth authentication. But it is also possible to add custom authentication directly to the request header.
Basic
Setting "Authentication" as Basic will use the current user for authentication, but you can also add named basic authentication to the system configuration. In that case, you would instead set that name as "Authentication". Named authentication must be used if you want to add mapped user credentials in OmniFi Admin.

OAuth
Configurations for OAuth authentication methods must be added to the system configuration before they can be used. More information can be found in the "Technical Whitepaper".
Once it has been added, all you need to do is to set its name as "Authentication".

The OAuth authentication flow typically directs you to an external web page where you can grant OmniFi access to the requested resource. After that, it will send an access token back to OmniFi through a callback. By default, the browser will block pop-ups and applications, so make sure to allow any pop-up and the OmniFi Authentication application.
Once you are authenticated, OmniFi will keep track of the access token and refresh it before it expires, provided that this is supported by the authority. Otherwise, you will be asked to authenticate again when it expires.
The number of active tokens can be seen on the About screen. Here it is also possible to remove them and stop any future refresh.
Header Authentication
It is possible to add any value to the request header, and that can also be used for authentication. For example, instead of using OmniFi Basic authentication, you can add the basic authentication token directly to the header.

REST Basics
The REST standard is widely used to create a stateless and reliable web API. It supports the HTTP methods GET, POST, PATCH, PUT and DELETE.
Resource Address
The address for a resource is typically in the format:
https://<host>/api/<resource_noun>/<resource_id>/<subcollection>
<resource_noun>
must always be included, while <resource_id>
and <subcollection>
are used depending on what resource you want to interact with.
It can also have query arguments in the format ?<field_1>=<value>&<field_2>=<value>
REST Methods
- GET - Read existing records. The result of the request is one or more record models.
- POST - Create new records. The record model is sent in the POST request.
- PATCH - Update existing record. The record values that should be updated are sent in the PATCH request. Other values will not be changed.
- PUT - Update existing record. A PUT request will, unlike PATCH, update the entire record. So the full record model should be sent in the PUT request. Other values that are not included will be removed.
- DELETE - Removes a record.
REST in Data Processing
REST API will use the normal data processing keywords that you are familiar with. These can be used to read, create, update and delete data. OmniFi uses JSON format for data sent to and from the web API.
Request URI
OmniFi will use the keyword value to define the format for the URI. It should always have the resource noun, but can also include arguments that are defined by [] in the Data Processing row.
So for example, if you want to query a resource /workspaces/1
you would use the following syntax:
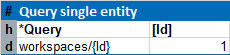
The URI format supports multiple arguments, allowing you to specify a URI for a sub-collection:

The URI format can also be used to handle query arguments. So if you for example want to query workspaces by name, you can use the following syntax:

Request Body
For POST, PATCH and PUT you would also have a body in the request. This would be a model that matches the resource definition. Header values without [] will add or update the current model that is stored in memory. That model would then be sent to the web API when the *Apply
keyword is used.
For example, the following syntax would create a model with Name that is sent to the web API.

The body in this case will be the following JSON text.
{
Name = "Test"
}
Request Header
The request header is a list of key/value pairs used to communicate various information to the service, for example accepted (accept:application/json
) or Authentication headers.
Custom request headers can be configured in the import service header by adding columns to the *Options
section. The following example adds a Version property to the request header:

Query a record (GET)
The *Query
keyword can be used to read data. And just like other services, [<query_key>] is used for query arguments.

*GetValues
can be used together with [response] to get the result from the last request.
Create a record (POST)
The *Create
keyword can be used to create a new object that can be posted to the API by calling *Apply
.

*GetValues
can be used together with [ToJSON] to get the current object.
Update a record (PATCH/PUT)
For PATCH updates where only the values sent in the request are updated, you should use the *Update
keyword to create a new object that is sent as a patch request on *Apply
.

For PUT updates where the full model is sent in the request, you should first use *Query
to read the current model that can then be updated and sent as a put request on *Apply
.

Remove a record (DELETE)
The *Remove
keyword can be used to send a delete request. Before calling *Remove
the model should be queried with the *Query
keyword.

Updated about 2 years ago